First, we need an area to start coding. After the project has been created, click “File” in the upper left hand corner and then click “NewFile”. The box below will pop up. Highlight “C” and then “C Main File” and finally click “Next”.
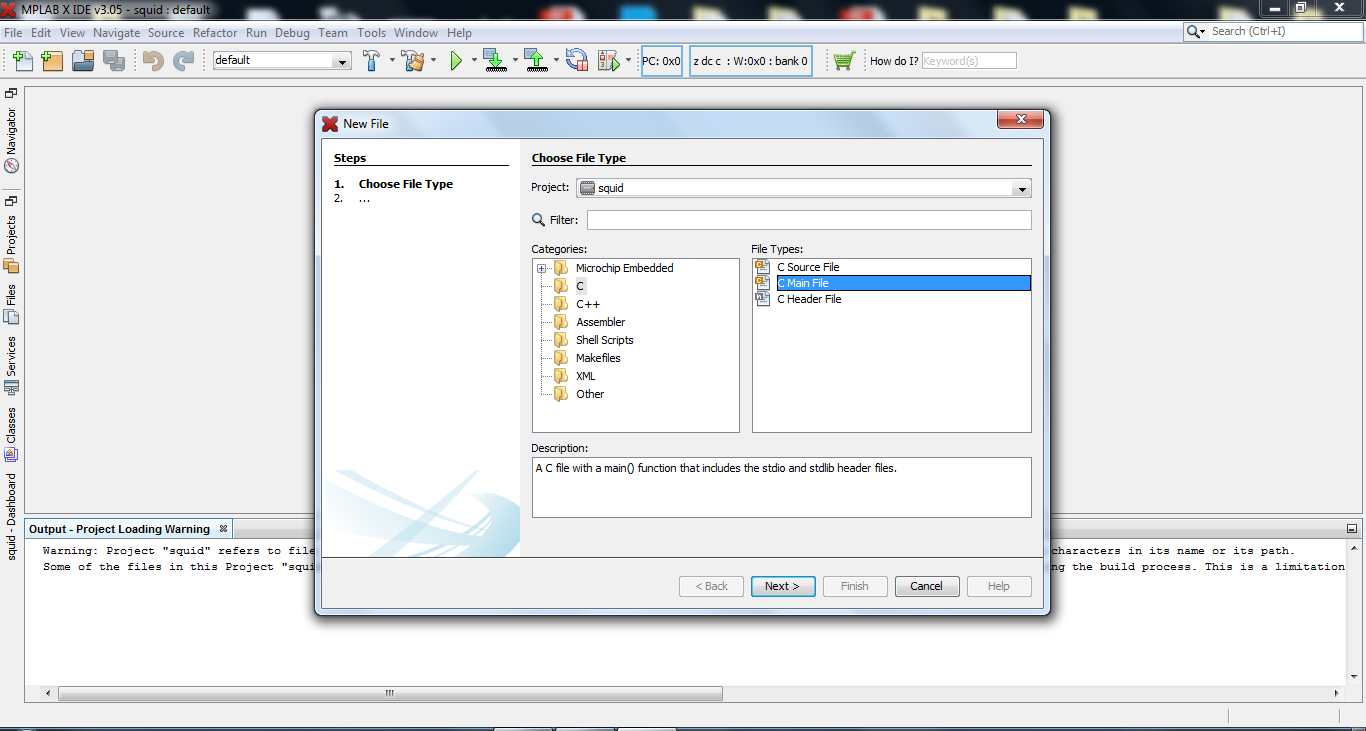
In the next box, you can name the page. I called mine sqmain. Then click “Next”.
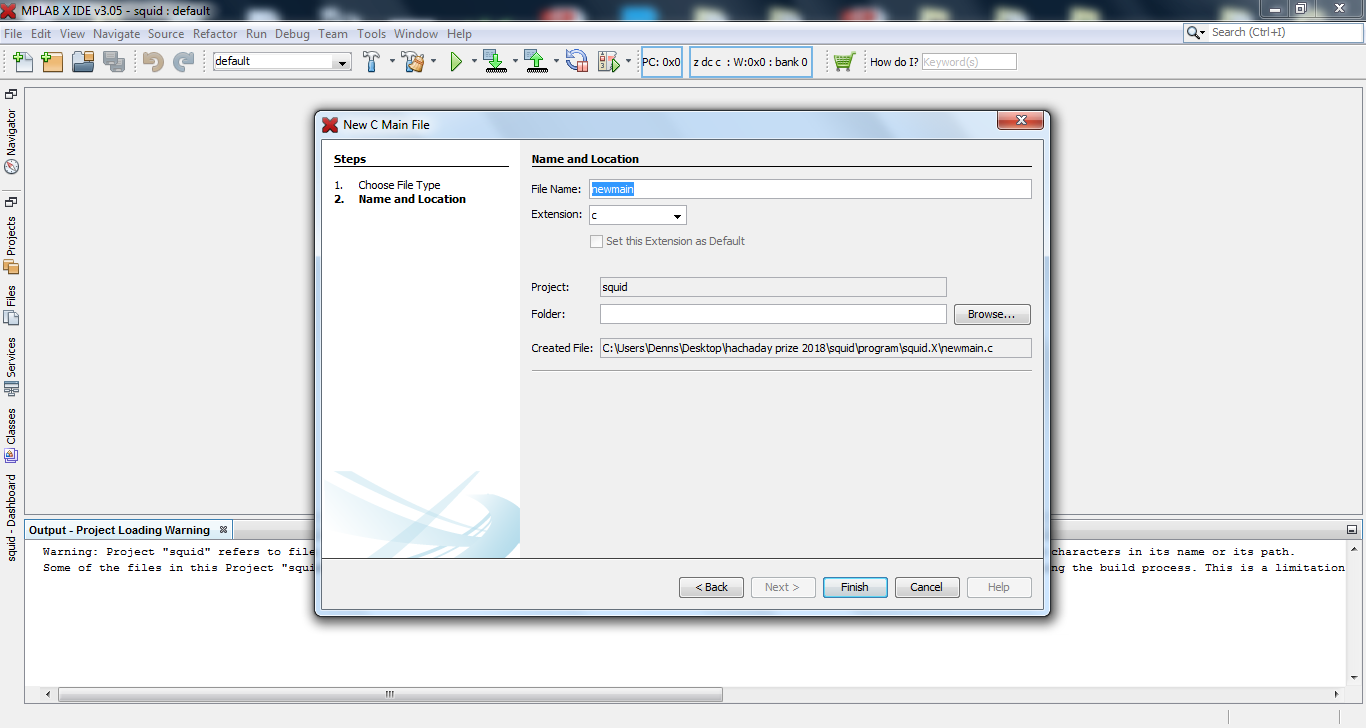
We now have a page to code on.
Next, include the h files. I also “defined” a crystal frequency “XTAL_FREQ” as 20 Mhz to be used in later time delays. Also define a couple of subs “void usart_setup(void);” and “void usart_write(char);”. Both sub-routines are self-explanatory. The setup sub-routine will be used to setup the PICs USART and the write will transmit the “char” data. I didn’t define a receive sub-routine because I will be using an interrupt to receive data. The configuration for the PIC is also shown below. I also created an array “char uart_receive_array[10];”to save data being received by the usart.
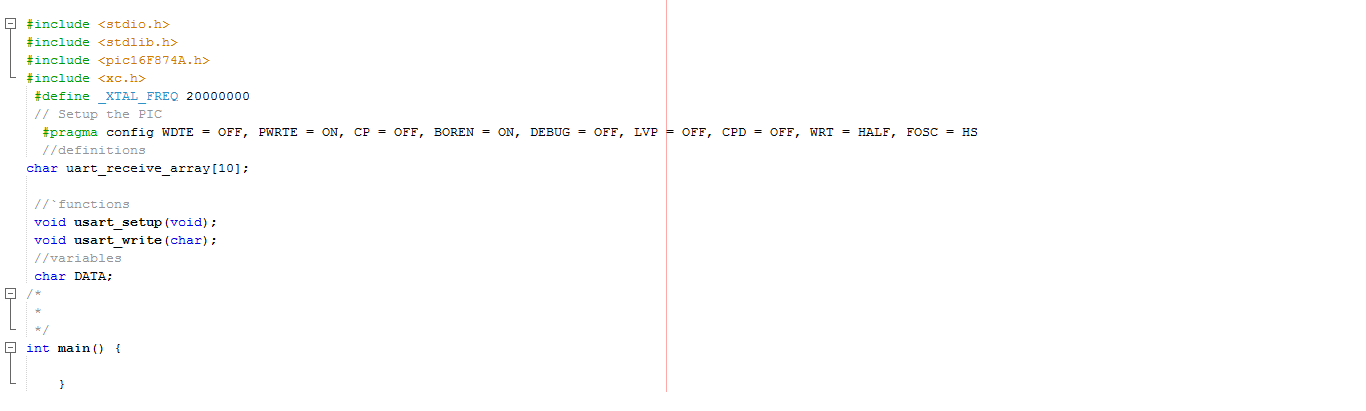
Below is the “usart_setup” sub-routine. I know I should set it up to calculate changing the buad rate, but for now, this will get the USART running. The buad rate is set to 9600 which is the Bluetooth module’s initial speed. The USART will be in asynchronous mode, and transmit and receive will be continuously enabled.
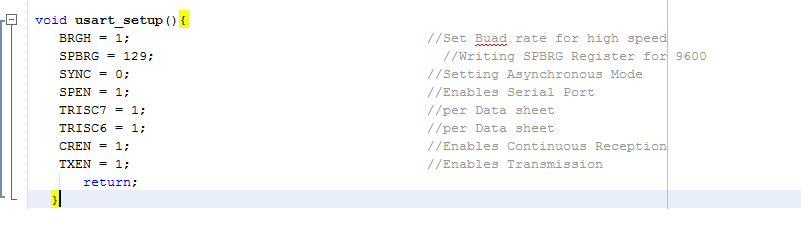
Below is the transmit sub-routine. Short and simple, call the sub with “usart_write('a');” and the usart will transmit an “a” and wait for it to complete.

Finally the interrupt. For now, just the usart receive interrupt is handled, but additional interrupts can be added as needed. When an interrupt is generated, the interrupt handler below will disable global interrupts “GIE = 0;” and check for the interrupt that was generated. If the USART receive interrupt was generated, each data register in the array will be moved to the next highest register. The lowest register “uart_receive_array[0]” will receive the new character being received. The character will then be sent to the write sub-routine “usart_write(uart_receive_array[0);” to acknowledge that the character has been received. I will be adding code to decode the received commands in future updates. Before exiting the interrupt handler, the global interrupts are re-enabled “GIE = 1;”.
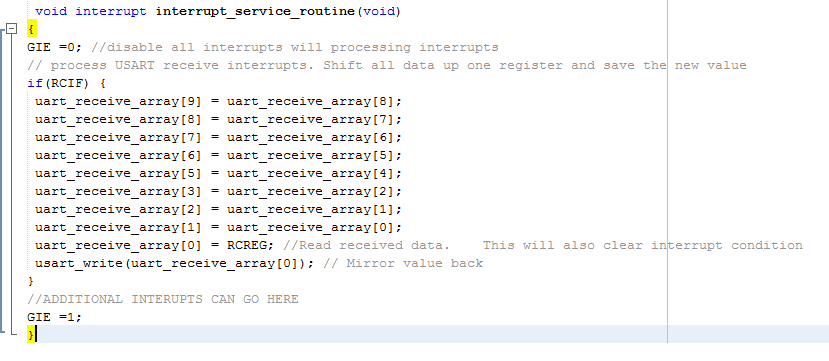
These bits will need to be set in the “main()” routine to enable the USART received interrupts.
RCIE = 1; //Enable interrupt on receive data
PEIE = 1;
GIE =1; //Enable interrupt
After testing, it seems to be working.
Discussions
Become a Hackaday.io Member
Create an account to leave a comment. Already have an account? Log In.