The Task
Okay, nice to have a task to guide the exploration. So let me build a "gCodeSender" clone for Linux. "gCodeSender" (https://wiki.shapeoko.com/index.php/GcodeSender) was my preferred Windows program for controlling my cheap Chinese laser plotter:
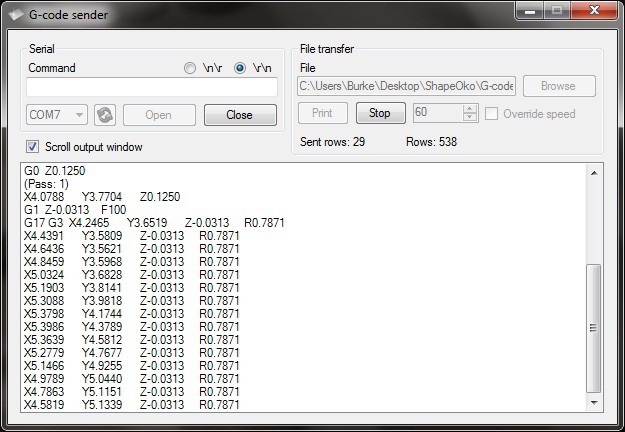
For this project I will need a serial library. "libSerialPort" seems to be the standard library that is available (for Mint: Synaptic Package Manager - libserialport-dev) else I could use RS-232 library (https://www.teuniz.net/RS-232/). I rather like the RS-232 library.
Before using these serial port libraries you may need set the permissions for your ports using:
- sudo usermod -a -G dialout $USER
and then
- login and logout
Grbl
"gCodeSender" talks to "Grbl" (https://github.com/grbl/grbl/wiki).
AlanX