Zenity
Zenity is a command line GUI that is probably already install on your Linux OS:
which zenity
Should return:
/usr/bin/zenity
If not you can install zenity with:
sudo apt-get update -y
sudo apt-get install -y zenity
Zenity Help
You can get help for Zenity by typing:
zenity --help
To get topics, then for specifics:
zenity --help-forms
Testing if Zenity Exists
Here are the top few lines of my Zenity test program where I test if Zenity is installed:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[])
{
char line[256];
FILE *fp;
// Test if Zenity is installed (get version)
fp=popen("zenity --version","r");
if (fp==NULL) {
perror("Pipe returned a error");
} else {
while (fgets(line,sizeof(line),fp)) printf("%s",line);
pclose(fp);
}
...
Pretty simple, open a pipe (popen()) and sent the command "zenity --version" via the pipe to the OS, and then read any output from zenity via the pipe, which should be the version number (in my case it was):
3.32.0
The pipe (i.e. fp) will return a NULL if not installed.
Is that it, let go!
No, not all results are via the standard output (i.e. stdout).
In the case of a button press, Zenity uses the return code, we can access the return code using:
WEXITSTATUS(pclose(fp))
Here is the code:
// Test Zenity Question Message Popup
fp=popen("zenity --question \
--title 'Confirm to Proceed' \
--width 500 --height 100 \
--text 'Proceed with Installation (Yes/No).' \
","r");
if (fp==NULL) {
perror("Pipe returned a error");
} else {
printf("Exit code: %i\n",WEXITSTATUS(pclose(fp)));
}
The return codes are 0 for "Yes" and 1 for "No". Here is the GUI:
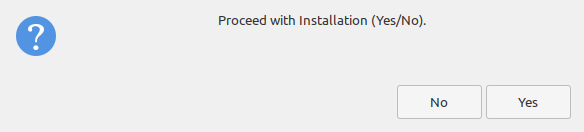
Something important to note:
Inside the double quotes (" ") I have use single quotes (' ').
This is the way C likes it, most Zenity examples are for shell scripts which don't mind inside double quotes, more double quotes..
Here is the code for a Form:
// Test Zenity Form Popup
fp=popen("zenity --forms \
--title='Add Member' \
--text='Member information' \
--separator=',' \
--add-entry='First Name' \
--add-entry='Family Name' \
--add-entry='Email' \
--add-calendar='Birthday' \
","r");
if (fp==NULL) {
perror("Pipe returned a error");
} else {
while (fgets(line,sizeof(line),fp)) printf("%s",line);
printf("Exit code: %i\n",WEXITSTATUS(pclose(fp)));
}
And the GUI:
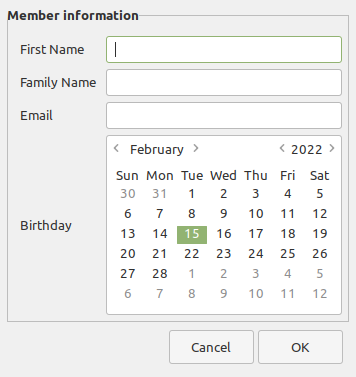
Adding Other Components
This where we discover limitations. I have added a Combo-Box for Sex and a List Selection:
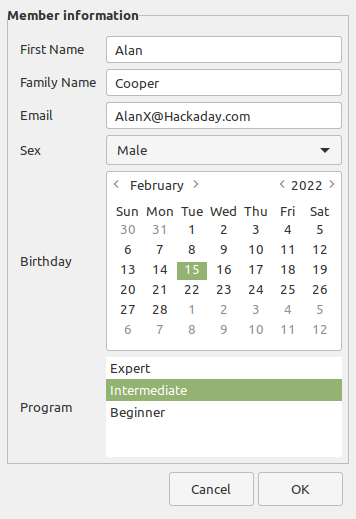
The main problem is there does not appear to be a way to initialise the Combo-Box or the List Selection. No big deal. There are other Widgets you can use but this is mostly all I need.
Here is the code:
// Test Zenity Complex Form Popup
fp=popen("zenity --forms \
--title='Add Member' \
--text='Member information' \
--separator=',' \
--add-entry='First Name' \
--add-entry='Family Name' \
--add-entry='Email' \
--add-combo='Sex' --combo-values='Male|Female' \
--add-calendar='Birthday' \
--add-list='Program' \
--list-values='Expert|Intermediate|Beginner' \
","r");
if (fp==NULL) {
perror("Pipe returned a error");
} else {
while (fgets(line,sizeof(line),fp)) printf("%s",line);
printf("Exit code: %i\n",WEXITSTATUS(pclose(fp)));
}
And here is the output:
Alan,Cooper,AlanX@Hackaday.com,Male,15/02022,Intermediate
and the exit code was:
0
which means "OK".
I hope you found this useful, AlanX