Update: Flip-disc displays will be available soon on Kickstarter.
The 1x7 flip-disc display project is a natural development of the 1x3 flip-disc display design. Here, as before, 595D shift registers were used to minimize the number of Arduino control lines.
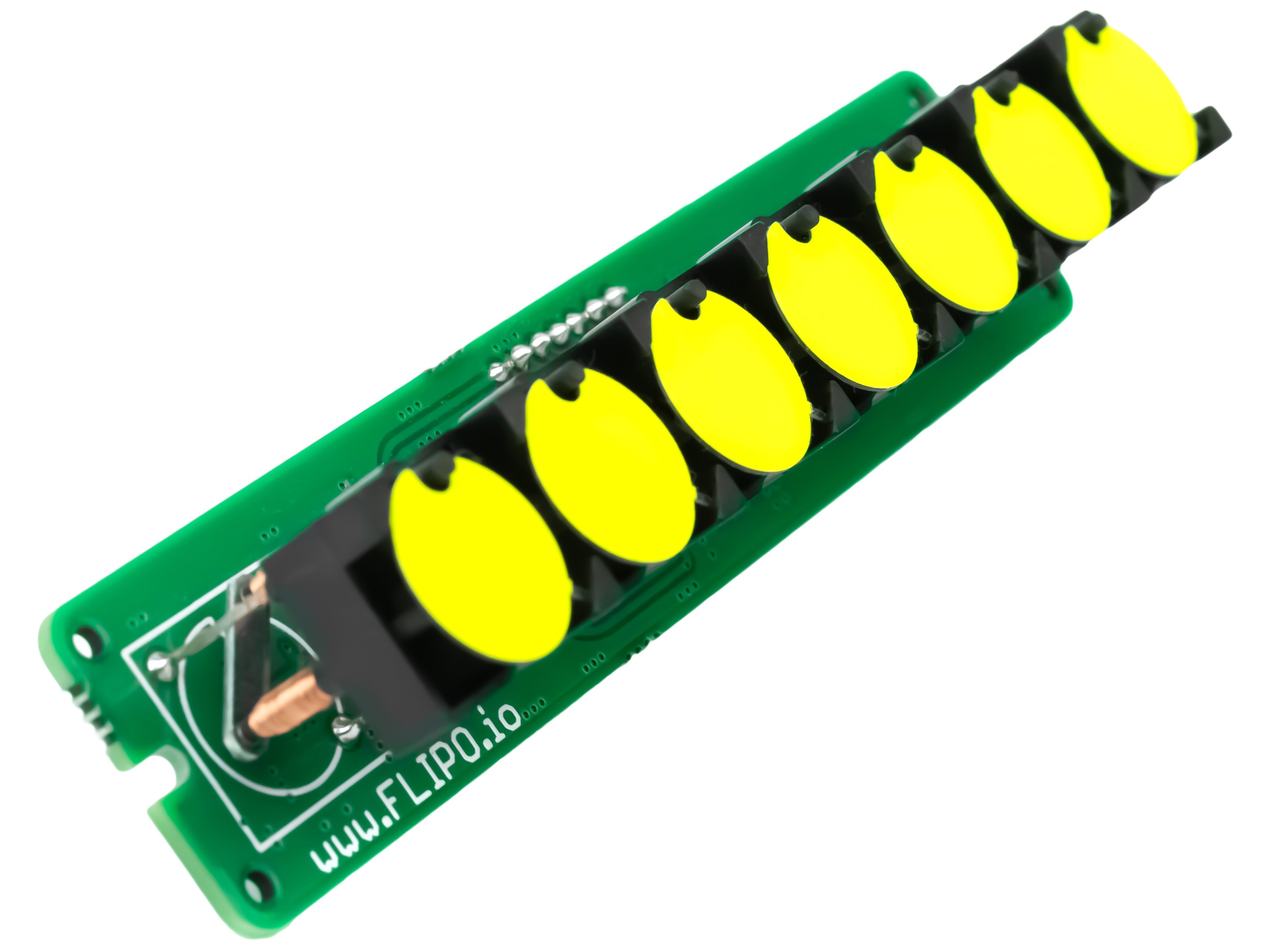
Flip-disc displays require a specific power supply, in order to flip the disc we need to supply the disc with a current pulse of about 0.5A with a precise length of about 1ms (see flip-disc datasheet) and with correct polarity.
The basics of controlling flip-disc displays and the principle of operation are described on the Flipo.io website dedicated to flip-disc displays and here.
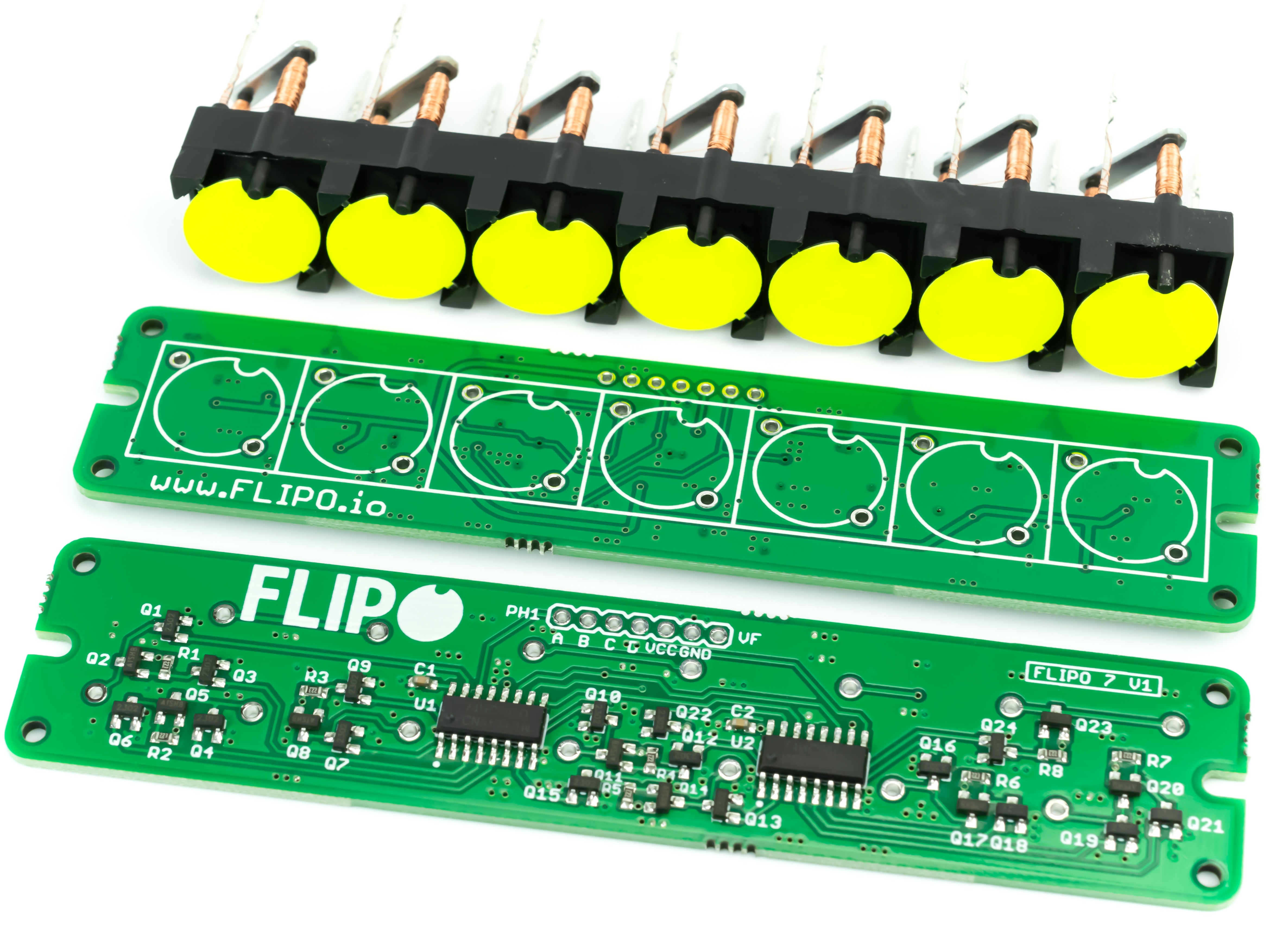
To control each of the disc, we will use the old tried-and-true method, the H-Bridge. We can also use ready-made H-bridge IC, but this method is cheaper, and its main advantage is that transistors are always available regardless of the turmoil in the electronics market.
Note:
Assuming that we have a power module, we can focus on the display design. It should be remembered that, this solution is prone to physical damage/burning of the transistors. If we mistakenly turn on both transistors on one side H1 & L1 or H2 & L2 at the same time, the transistors will be short-circuited and damaged. Therefore, you must be absolutely sure that the control code is resistant to errors that can lead to damage to the controller.
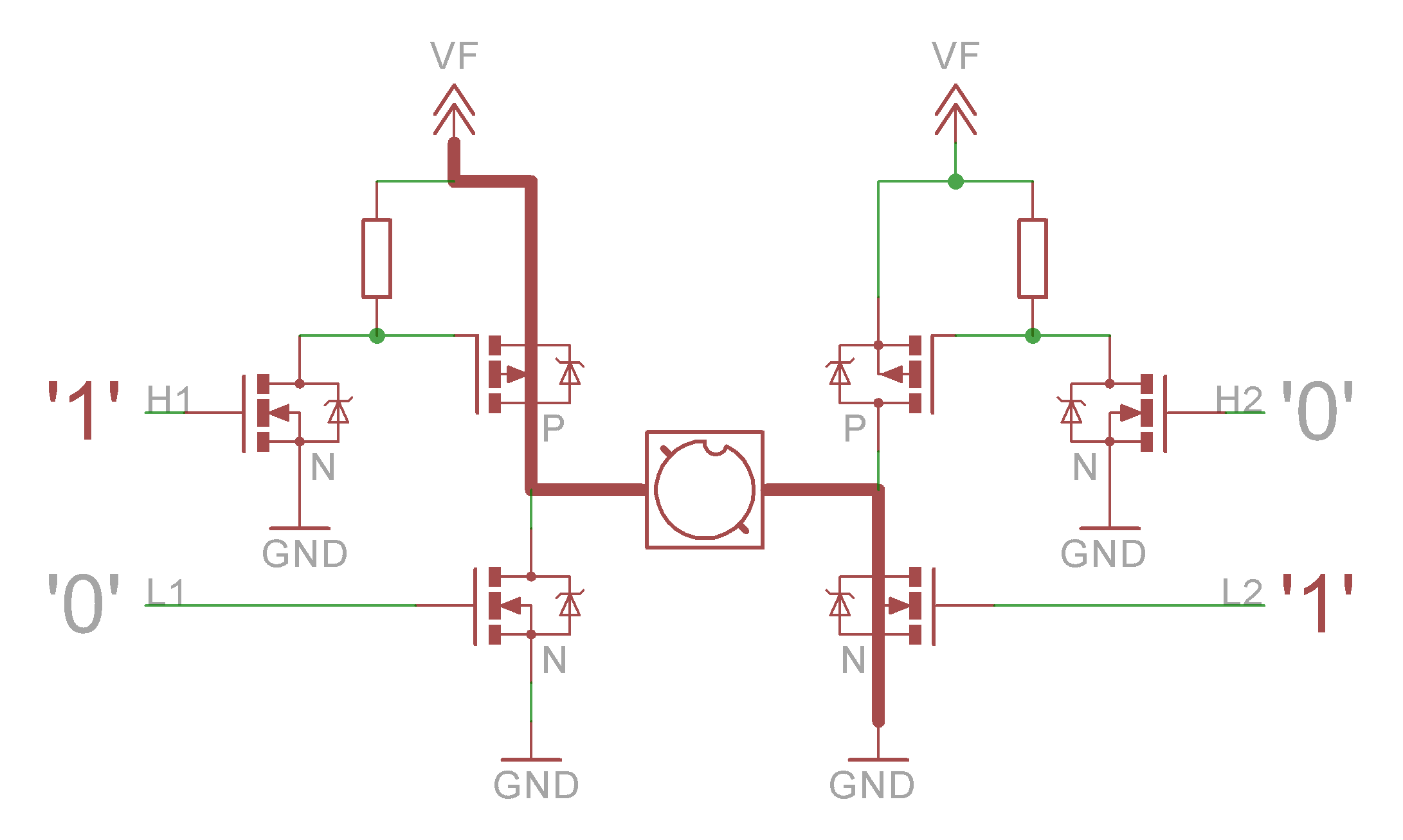
For simplicity, we can connect all the leads of the discs on one side to each other. Such simplification forces the control of single discs at a time, but remembering that the current pulse has a length of 1ms this limitation to one disc does not matter.
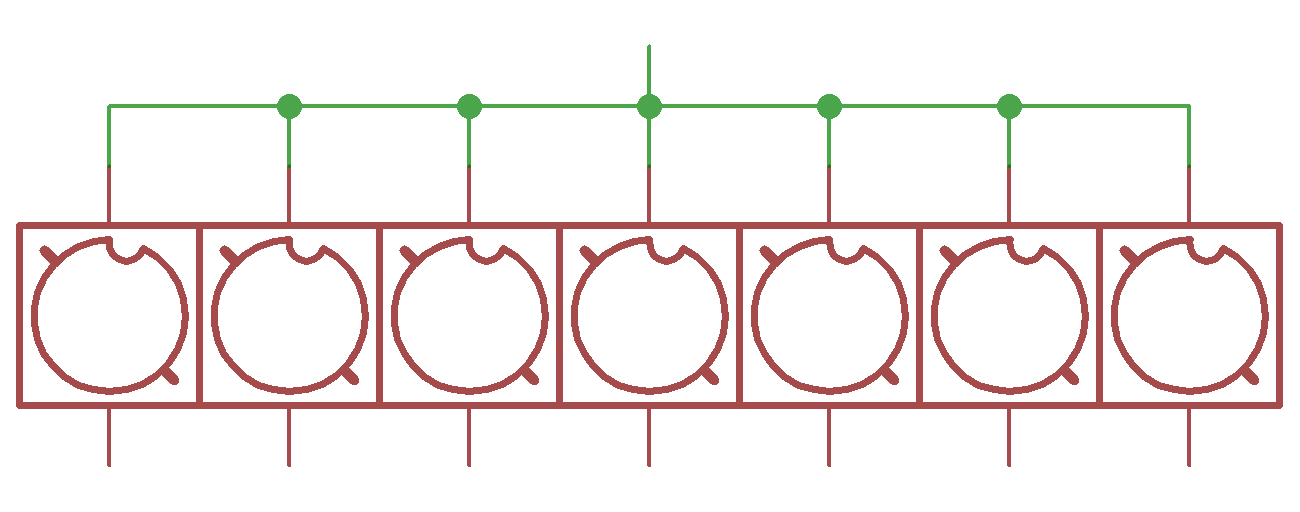
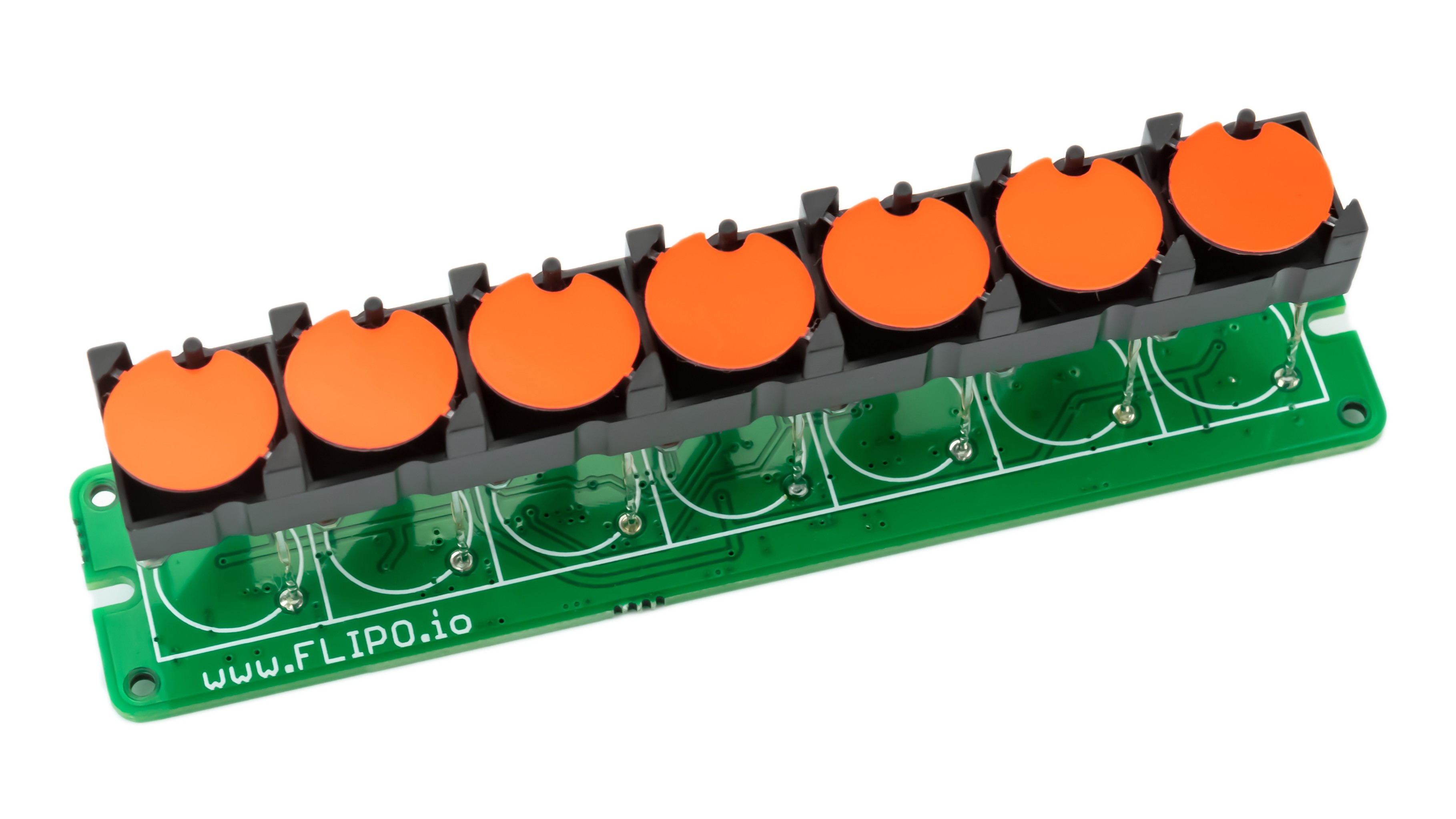
After simplification, we have 8 lines to be driven by H-bridges, which gives a total of 16 inputs. Controlling directly from the Arduino is not a very good idea, as there won't be too many free pins left for other Arduino tasks. The simplest way to control so many lines is to use shift registers.
An example scheme could look like this:
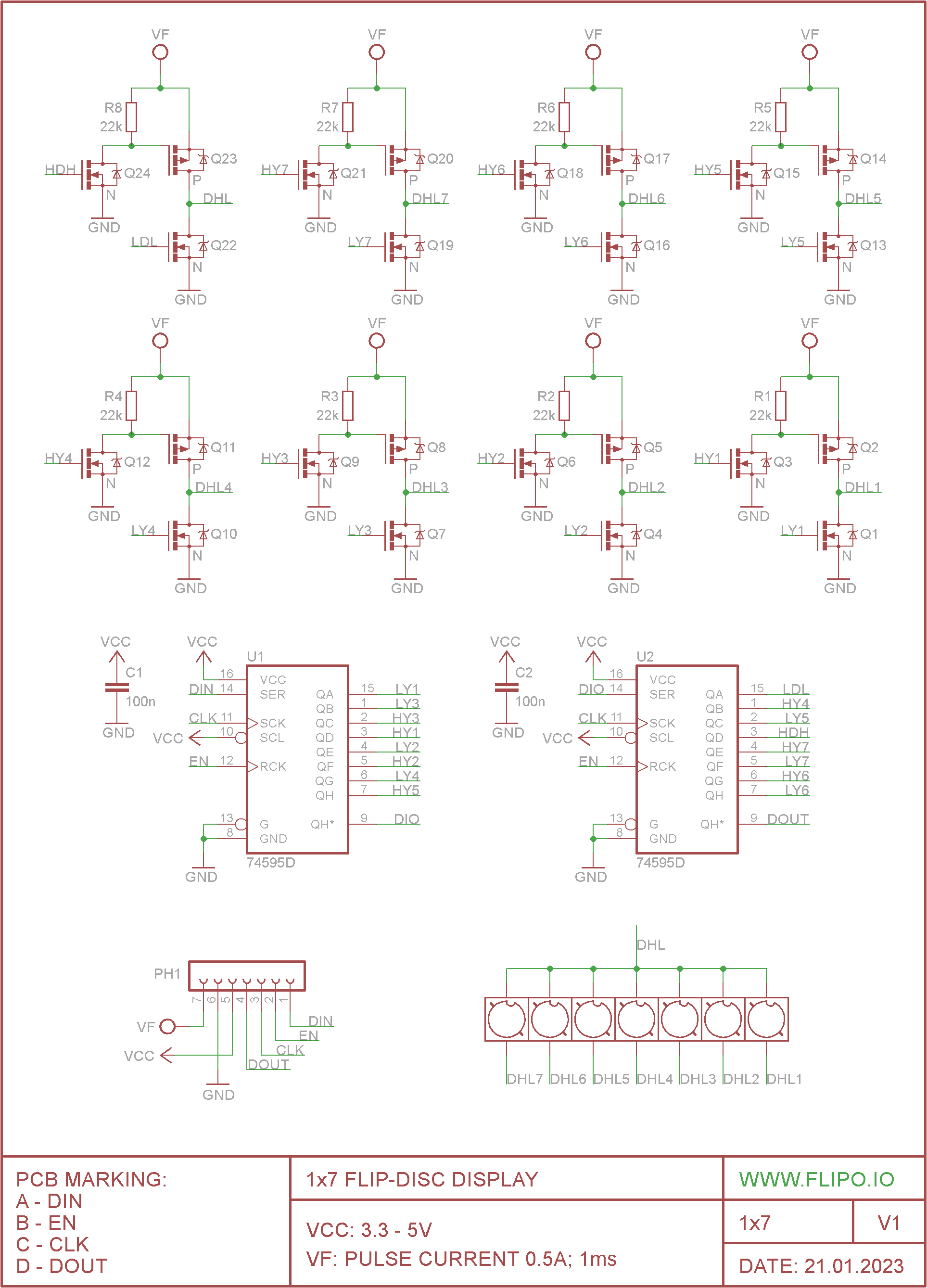
Only 3 control lines DIN, EN, CLK. For control, we can use standard libraries for 595D shift registers or use a dedicated Arduino library for Flip-disc displays. The library for display control uses SPI and requires connection of lines: DIN -> MOSI, CLK -> SCK, EN - to any digital pin.
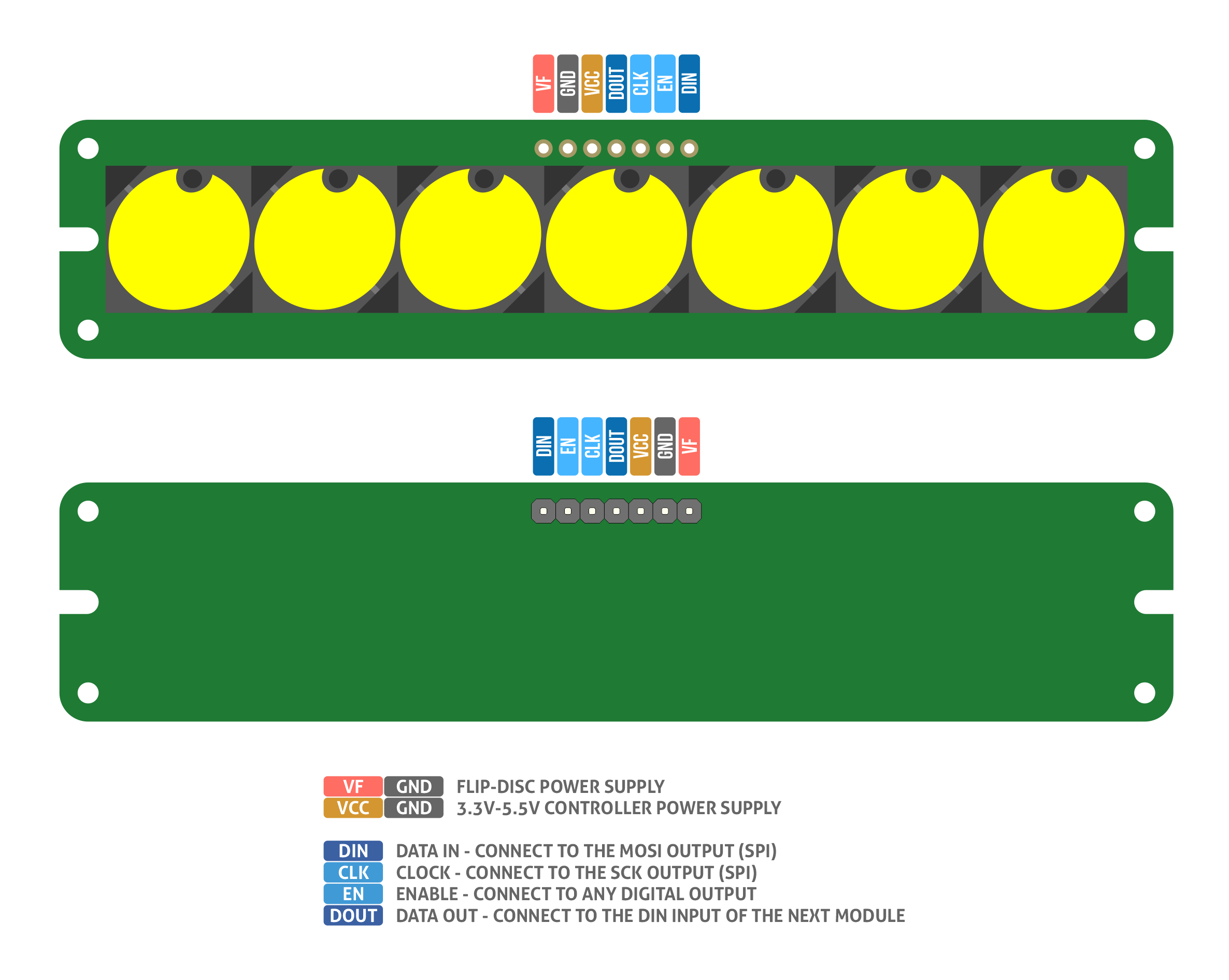
Note:
For proper operation of the display as mentioned above, a dedicated power module is required - a description of how it works can be found on the project page.
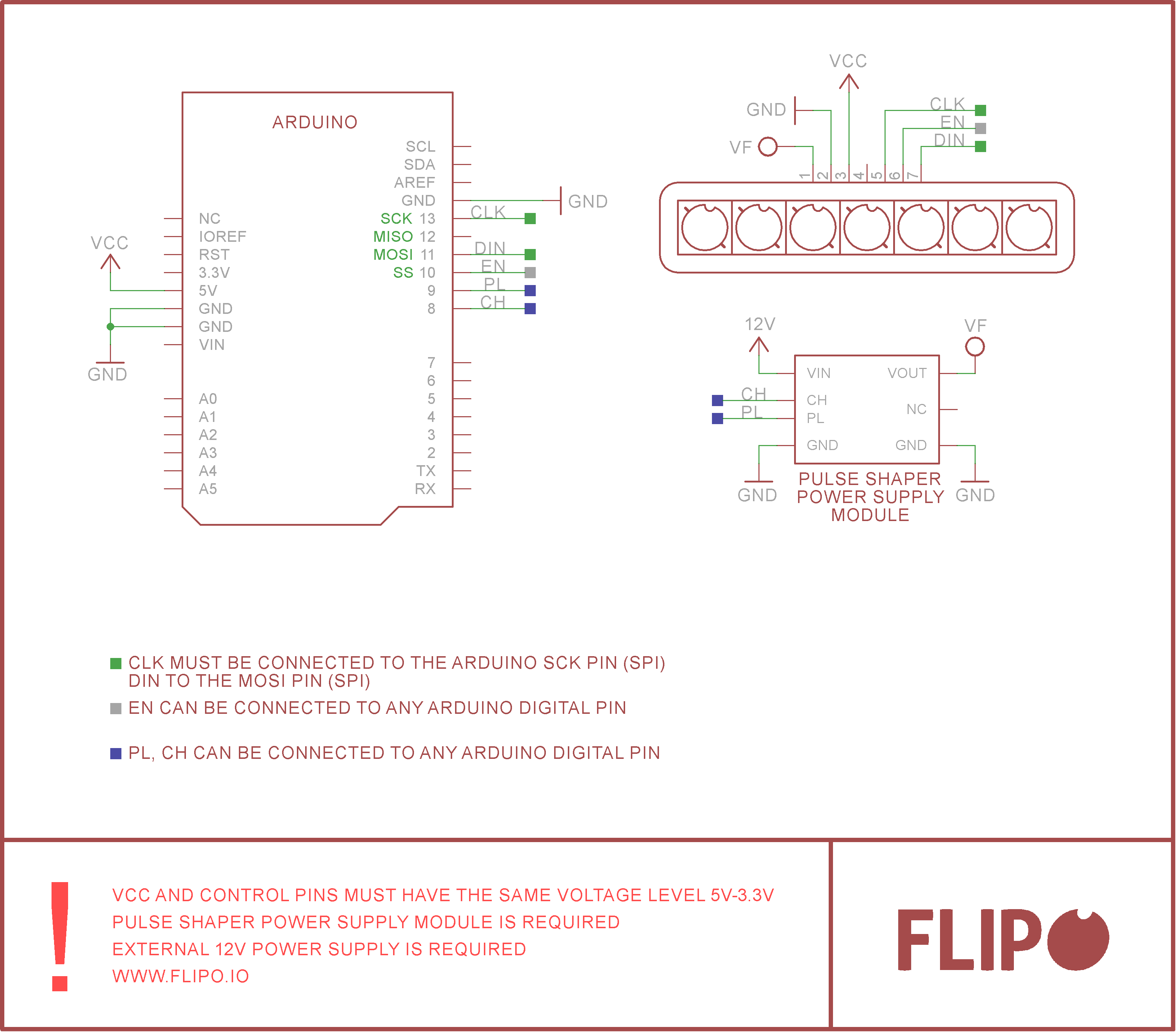
For such connection of modules as above, the display control code will look like this:
#include // https://github.com/marcinsaj/FlipDisc
// Pin declaration for Arduino Uno and PSPS module
#define EN_PIN 10 // Start & End SPI transfer data
#define CH_PIN 8 // Charging PSPS module - turn ON/OFF
#define PL_PIN 9 // Release the current pulse - turn ON/OFF
void setup()
{
/* Assignment of control pins */
Flip.Pin(EN_PIN, CH_PIN, PL_PIN);
/* Display initialization */
Flip.Init(D1X7);
delay(3000);
}
void loop()
{
/* The function allows you to control up to three discs of the selected display.
Flip.Display_1x7(module_number, disc1,disc2,disc3,disc4,disc5,disc6,disc7);
- module_number - relative number of the D1X7 display
- disc1, disc2,... disc7 - counting from left to right 1-7
For a detailed description of all display control functions,
see the library description: https://github.com/marcinsaj/FlipDisc */
/* Set all discs */
Flip.Display_1x7(1,1,1,1,1,1,1,1);
delay(1000);
/* Reset selected discs */
Flip.Display_1x7(1,1,0,1,0,1,0,1);
delay(1000);
}
More detailed code, along with many other functions that control the flip-disc display, can be found here and in the description of the Arduino FlipDisc.h library.
The schematic of the 1x7 flip-disc display contains one small detail, it is the DOUT output of the shift register. This output is used to connect another flip-disc display - DOUT output needs to...
Read more »