There was an attempt to manually upgrade some of the broken python dependencies.
python3 -m pip install 'onnx>=1.8.1'
All pip commands failed with a broken protobuf dependency. You can get all the dependencies from
https://pypi.org/project/protobuf/4.21.1/#files
https://pypi.org/project/onnx/1.8.1/#files
& manually install them with python3 setup.py install
OPENBLAS_CORETYPE=CORTEXA57 polygraphy surgeon sanitize efficientdet_lion.onnx --fold-constants -o efficientdet_lion2.onnx
Another go with this command worked.
/usr/src/tensorrt/bin/trtexec --onnx=efficientdet_lion2.onnx --saveEngine=efficientdet_lion.engine
Still threw
IShuffleLayer applied to shape tensor must have 0 or 1 reshape dimensions: dimensions were [-1,2]
The next hit was a very cryptic replacement of the pad operator with cat statements.
# x = F.pad(x, [left, right, top, bottom]) # add 0 to sides x = torch.cat((torch.zeros((n, c, h, left)).to(x.device), x, torch.zeros((n, c, h, right)).to(x.device)), dim=3) x = torch.cat((torch.zeros((n, c, top, w + left + right)).to(x.device), x, torch.zeros((n, c, bottom, w + left + right)).to(x.device)), dim=2)
cat takes a tuple of a bunch of matrixes to concatenate. The general idea is they're adding 0's to the left, right, top & bottom of an image. The X & Y axis is dimension 3 & dimension 2 of the image. There's no explanation of which side of the tuple is left, right, top or bottom.
This failed with
Assertion failed: (axis >= 0 && axis <= nbDims) && "Axis must be in the range [0, nbDims].
The ONNX modifier showed the offending operator getting a 4 axis input. Doing it in 4 cats instead of 2 yielded the same error. Interestingly, the polygraphy surgeon sanitize command expands 2 cat statements to 4.
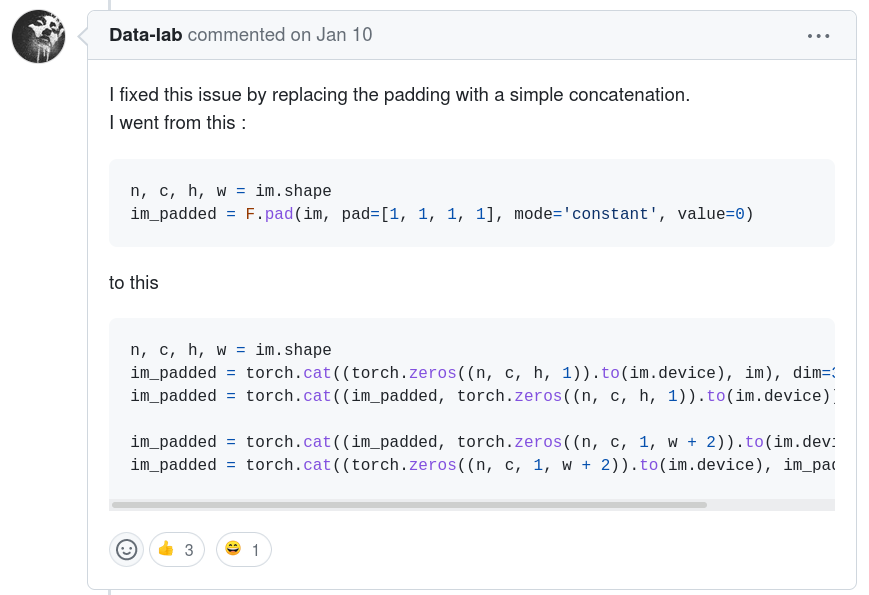
The number of likes for the offending answer makes lions wonder if anyone actually tried the code or if they're just studying for job interviews. All forums related to tensorrt die off in Feb 2023, right when chatgpt got big.
Chatgpt showed a way to copy a small matrix to an offset in a bigger matrix. They specify a destination range for each axis.
padded = torch.zeros((n, c, h + top + bottom, w + left + right)).to(x.device)
padded[0:n, 0:c, top:top+h, left:left+w] = x
x = padded
This actually got past the padding step & failed on a reshape step.
[6] Invalid Node - Reshape_1315
[graphShapeAnalyzer.cpp::analyzeShapes::1285] Error Code 4: Miscellaneous (IShuffleLayer Reshape_1315: reshape changes volume. Reshaping [1,96,160,319] to [1,96,160,79].)
The chances of all these workarounds working without any way to incrementally test were pretty small. At this point, lions wondered what anyone ever used the jetson nano for if it couldn't import a basic object recognizer or if this efficientdet model was a dead end. Maybe everyone used the FP32 version inside pytorch to learn the concept but no-one bothered optimizing it for tensorrt. It was pure luck that body_25 ported to tensorrt & the face recognition was ported by someone else.
The next hit was a conversion of efficientdet-lite to FP16:
https://github.com/NobuoTsukamoto/tensorrt-examples/blob/main/cpp/efficientdet/README.md
The goog previously couldn't find any efficientdet-lite model except INT8, so this was another step to try.
Discussions
Become a Hackaday.io Member
Create an account to leave a comment. Already have an account? Log In.