Want to know exactly how far away a DumDum is? I took the next step and wired in a 16 x 2 LCD that displays the distance in cm. It took a while to figure out the wiring for the LCD, but here it is. And the appropriate code too.
Code:
#include <LiquidCrystal.h> LiquidCrystal lcd(12, 11, 5, 4, 3, 2); int ledRed = 9; int ledGre = 6; int ledYel = 7; #define trigPin 10 #define echoPin 13 void setup() { pinMode(trigPin, OUTPUT); pinMode(echoPin, INPUT); pinMode(ledRed, OUTPUT); pinMode(ledYel, OUTPUT); pinMode(ledGre, OUTPUT); lcd.begin(16, 2); } void loop() { float duration, distance; digitalWrite(trigPin, LOW); delayMicroseconds(2); digitalWrite(trigPin, HIGH); delayMicroseconds(10); digitalWrite(trigPin, LOW); duration = pulseIn(echoPin, HIGH); distance = (duration / 2) * 0.0344; lcd.clear(); lcd.print("Distance = "); lcd.print(distance); lcd.print(" cm"); delay(100); if (distance <=400) { tone(8, ((150 - distance) * 10)); } else { tone(8, 0); analogWrite(ledGre, LOW); analogWrite(ledYel, LOW); analogWrite(ledRed, LOW); } if (distance >= 60 && distance < 200){ analogWrite(ledGre, 350); } else { analogWrite(ledGre, LOW); } if (distance > 30 && distance < 60){ analogWrite(ledYel, 600); } else { analogWrite(ledYel, LOW); } if (distance <= 30){ analogWrite(ledRed, 350); } else { analogWrite(ledRed, LOW); } delay(distance * 3); }Hardwares:
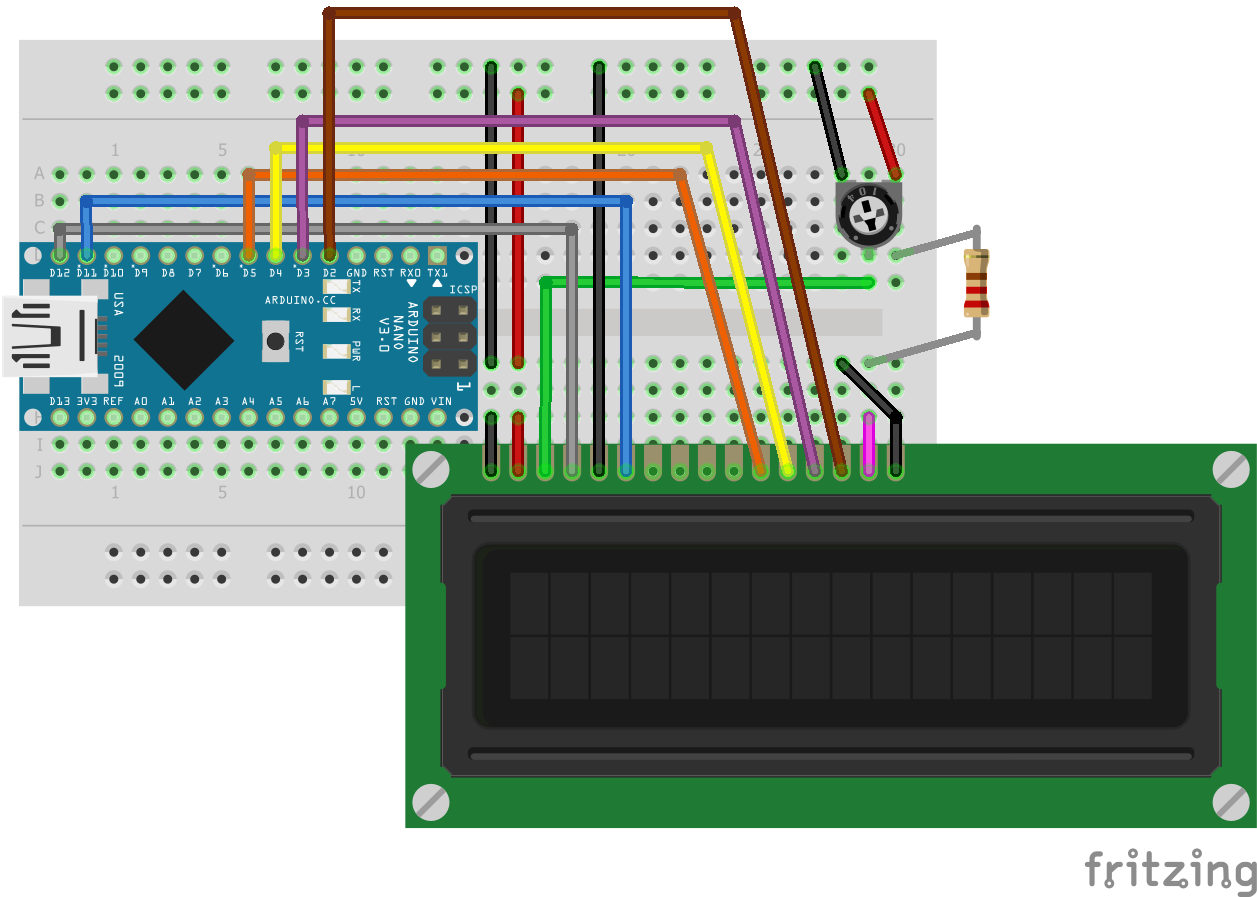
Just a note: That resistor is a 220k and the potentiometer is a 10K...
*Please note: These fritzing diagrams are intended to show only the proper connections, not necessarily the proper routing of components and wires... Bear this in mind if you are trying to replicate this build.*
Discussions
Become a Hackaday.io Member
Create an account to leave a comment. Already have an account? Log In.