This project derived from the x-ray machine. The addition to the machine are the motor to rotate the object and the software. The software is working already but needs finishing and will be uploaded here when completed. *Edit* source code here.
The program is written in C++ and it only uses OpenCV as external lib.
I have done some testings to it, here you can see a lighter:
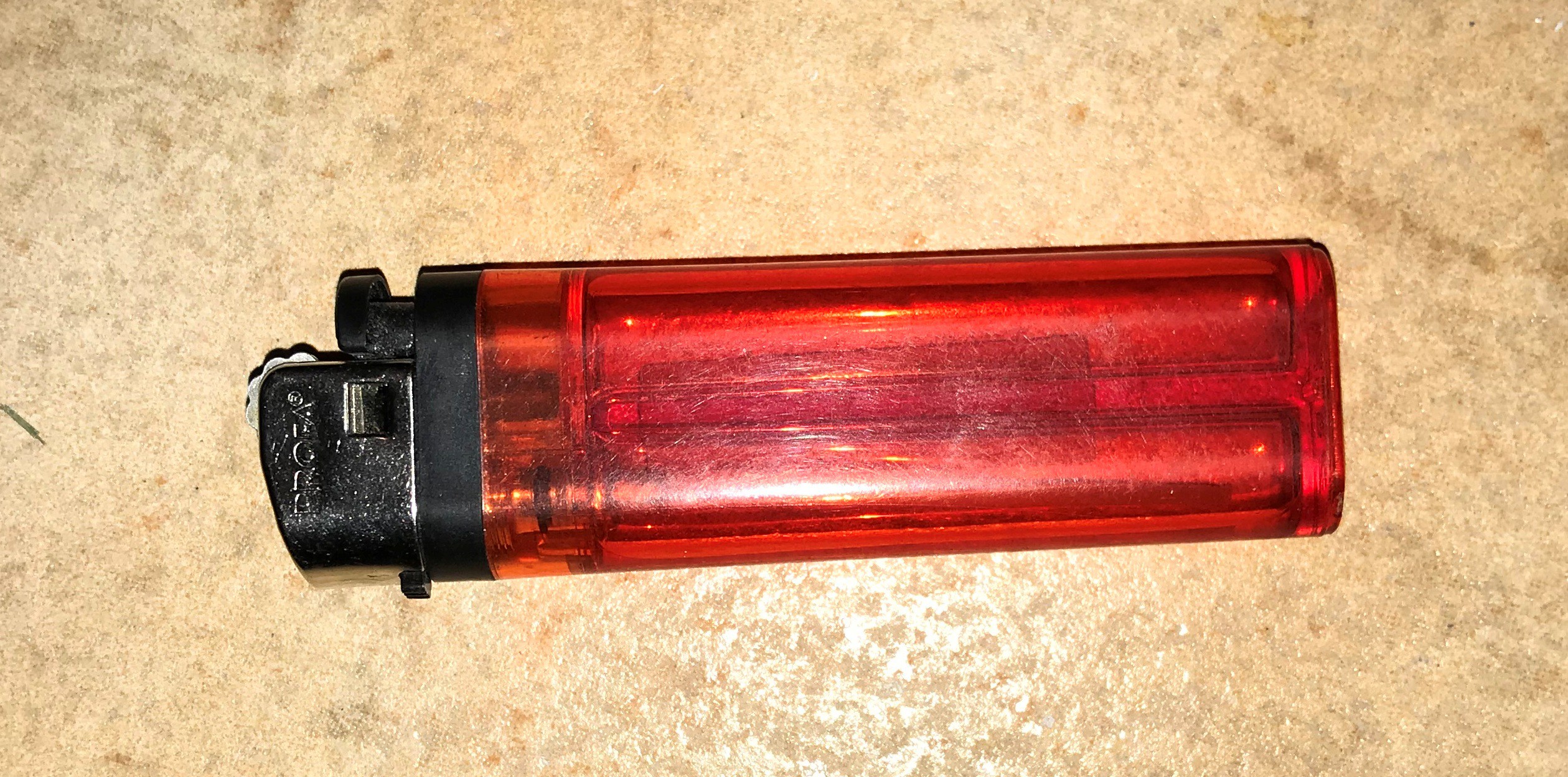
You can see it spinning opening the file "lighter.mp4"
The program then performs the reconstruction. This is the axial view of a slice:
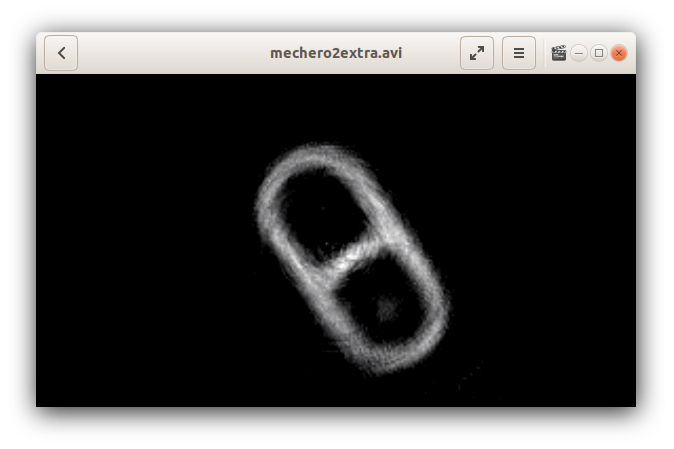
The program also saves the object in point cloud format so you can open it with a 3D point cloud viewer:
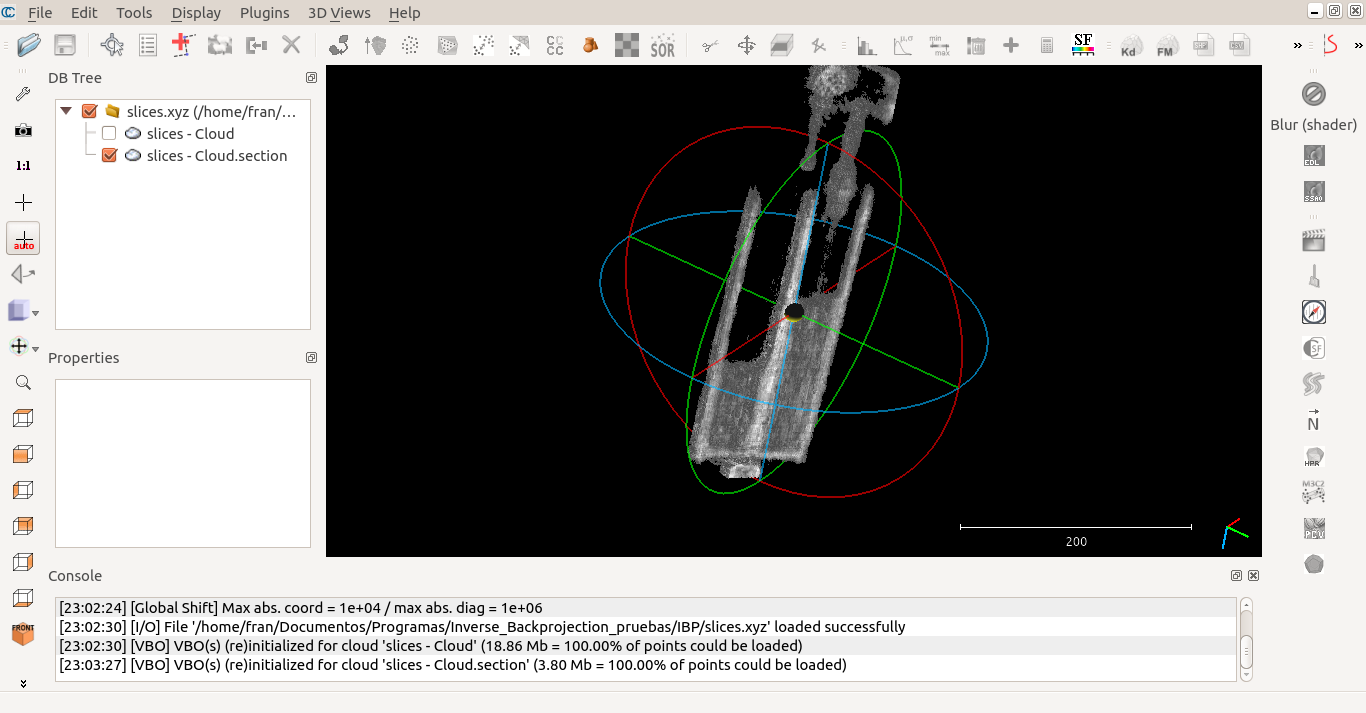
The cool thing about this is that you can cut out the walls in the computer and separate parts, above you can see that I removed the walls of the lighter and I'm now able to see the gas.
Another test this time with a GU10 LED lamp (see file "led.mp4"):
and the reconstruction:
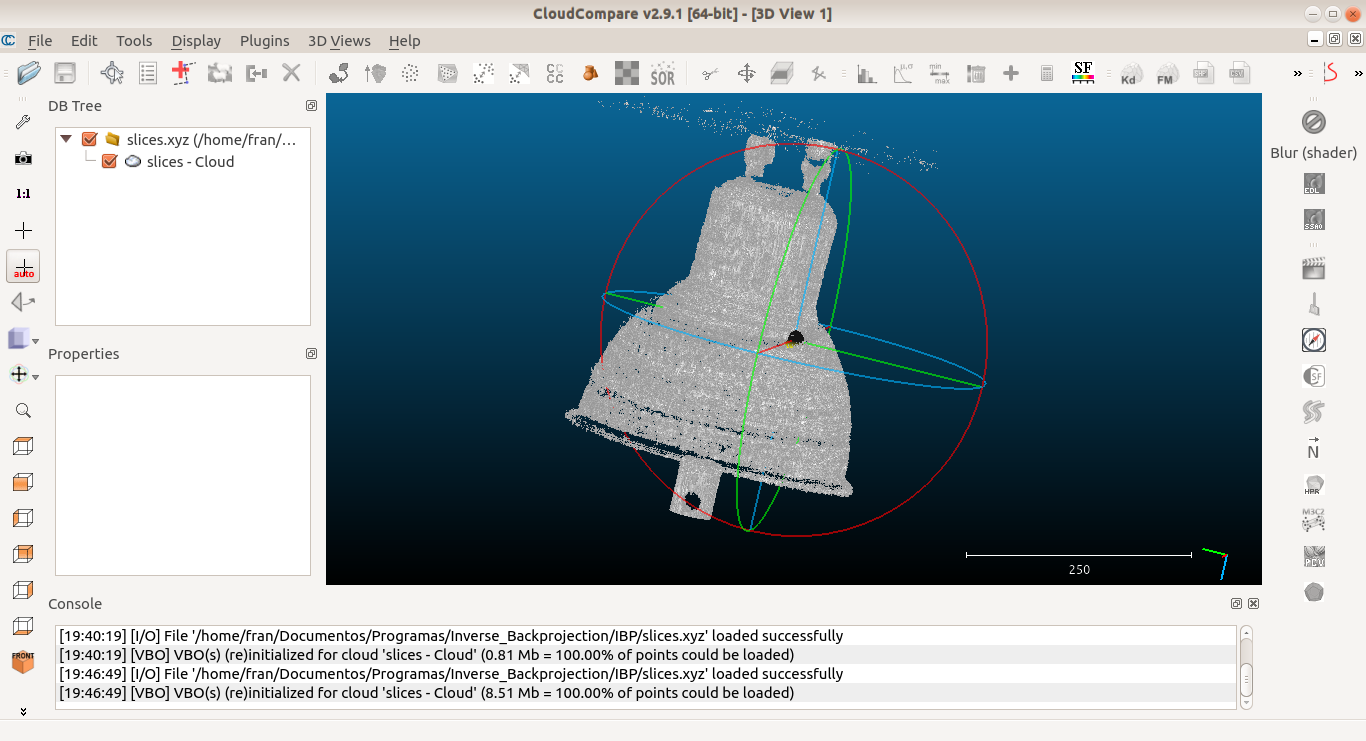
The program computes all slices, so I can see how many leds the lamp has, by selecting the proper slice:
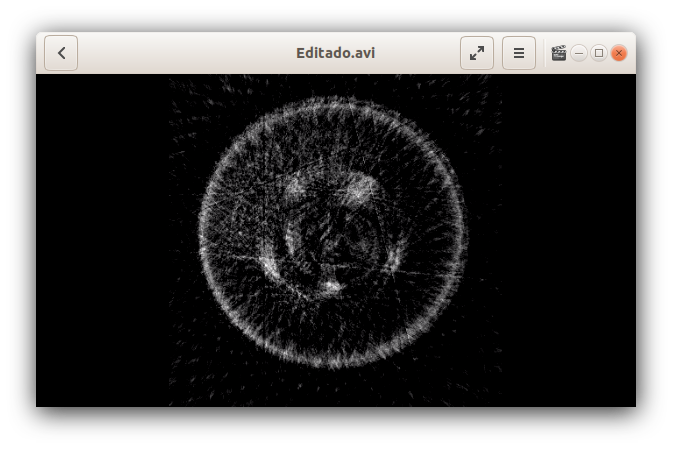
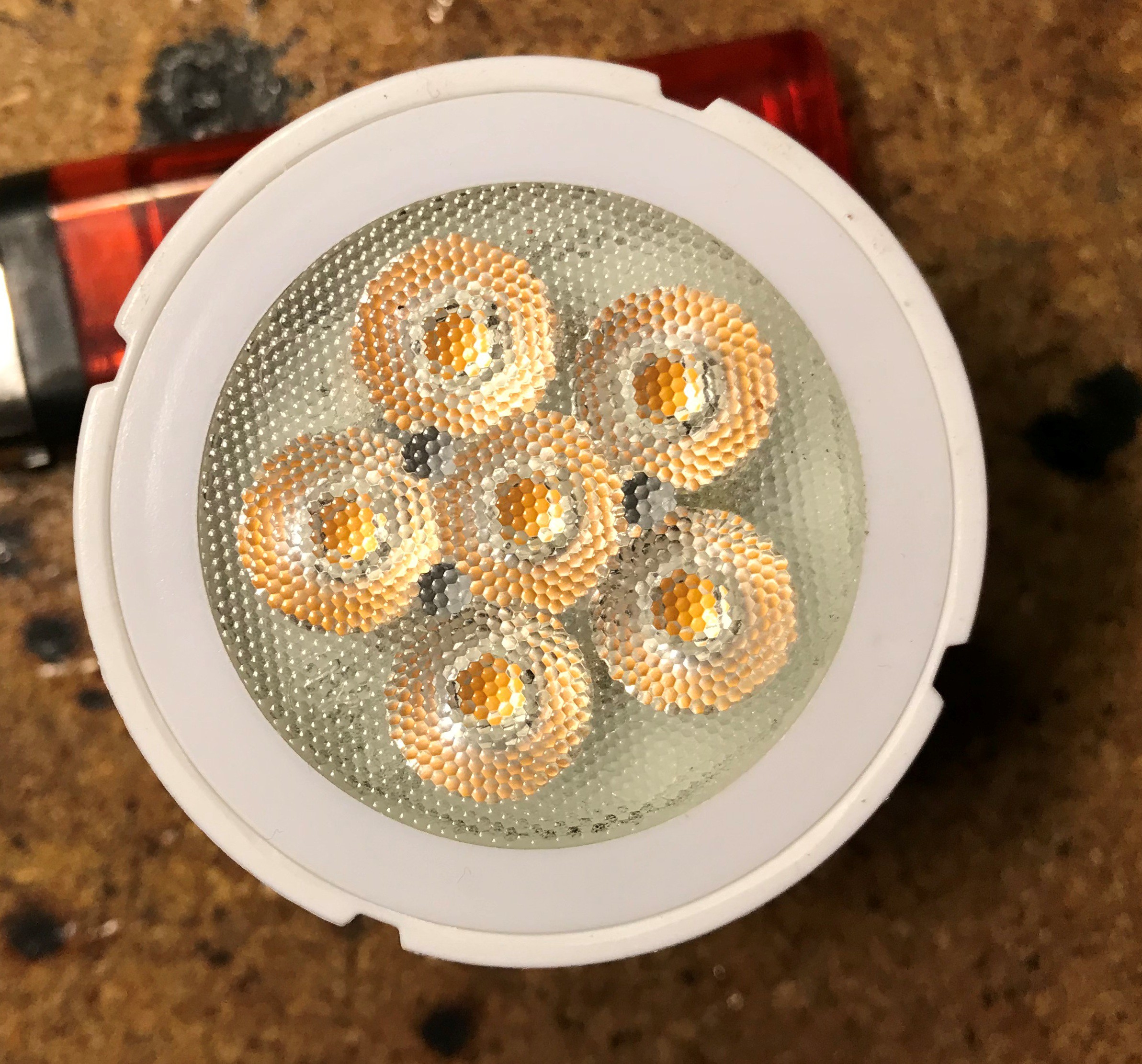
Well, not bad keeping in mind that I forgot to turn off the laser grid, so I have lots of artifacts coming from it.
Now I will explain how the program works:
- First I record a video of the object spinning, only for around 3 seconds. My camera records at 25fps so that means very few images to do a reconstruction free of noise, but we will see later that It's not a big problem.
- I then pass the video to the program. It loads each frame of the video in a vector of (OpenCV) Mat objects.
- The program asks me to select wich frame is the beginning (angle 0) and ending (angle 360) of the scan.
- The program removes all frames except these and then asks me to select the object:
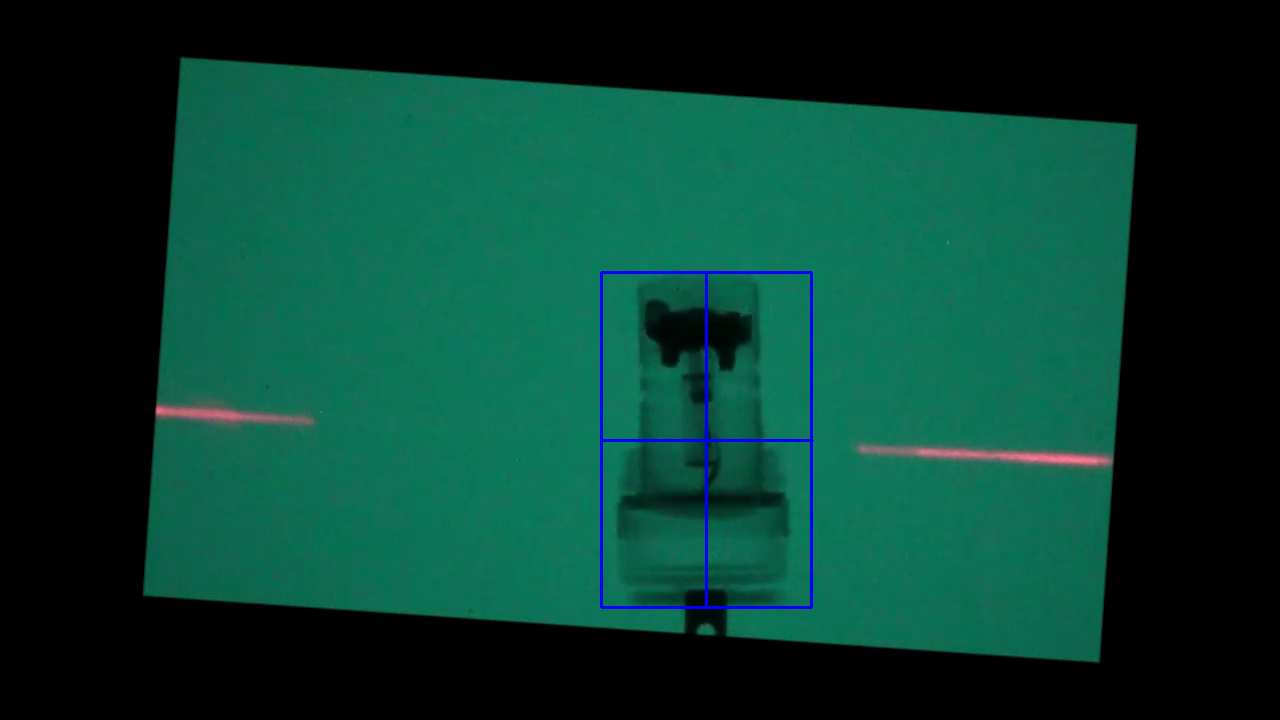
- I select it in a way that the vertical blue line of that grid matches the axis of rotation.
- The program converts the images from 8bit BGR to 32bit RGB, with pixel values normalized to 1.
- A gamma correction is applyied to all channels of all frames, this is done just taking each pixel and raising it's value to the power of 2.2.
- The program now performs a linear interpolation between frames, adding 1 extra frame for each pair of frames recorded, so I now have doubled the number of images I have. This has the effect that the reconstructions are a bit blurry but the noise has almost gone completely.
- All images are converted from 32bit RGB to 32bit grayscale. My scintillation screen glows green so I'm just taking the G channel for this.
- The program ask the user to select an area corresponding to the background. This is done to know how bright is the x-ray beam and then a new correction is done, all pixels get a new value known as absorption:
- The program makes the sinograms. We will have as many sinograms as height in pixels of the input frames of the object.
- All sinograms get a high pass filter.
- Now the inverse Radon transform is calculated for all sinograms and we will get all the slices.
- The program saves the slices in point cloud .xyz format and also in video format, yo can see all the slices of the lighter opening the file "ligher_slices.avi". You will see all slices in axial view, starting from the top ang going down.
And that's it, many thanks to user nevermore78 for helping me with the image processing :D
Amazing project! What are you using for the scintillation screen? Is it something you made?